In TypeScript and Javascript, closures are a powerful concept used to manage data and scope within functions. However, sometimes closures can cause unexpected issues when working with asynchronous operations and React components. One such problem is known as “stale closures,” and it can significantly affect the behavior of React components. In this article, we’ll explore what stale closures are, why they occur, and how they can impact React components.
What is a Stale Closure?
In simple terms, a closure is created when a function “remembers” its surrounding environment, even after the function has finished executing. Stale closures occur when this remembered data becomes outdated due to the asynchronous nature of JavaScript and TypeScript. This commonly happens in scenarios involving data fetching or timers within React components.
Why Do Stale Closures Occur?
Stale closures arise because of the asynchronous execution of code. When an inner function references variables from its outer scope, it retains a connection to those variables. If the variables change before the inner function runs, the closure holds onto outdated data, leading to unexpected outcomes and bugs.
In the context of React components, stale closures often happen when using hooks like useState ,useEffect , useMemo, and useCallback. When an effect captures variables from the component's scope and those variables change, the effect might still rely on the old values, causing issues in the component's state management and rendering.
Impact on React Components:
Stale closures can have various effects on React components, including:
- Incorrect Rendering: Stale closures may cause components to render incorrect data. When a component uses stale data from a closure, it could display outdated information, resulting in a mismatch between the displayed UI and the actual application state.
Example Code:
import React, { useState, useEffect } from "react";
const IncorrectRendering: React.FC = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const intervalId = setInterval(() => {
// Incorrect: Stale closure capturing 'count' which is always 0
console.log("Count", count);
setCount(count + 1);
}, 1000);
// Clean up the interval on component unmount
return () => {
clearInterval(intervalId);
};
}, []); // Empty dependency array to run the effect only once on mount
return (
<div>
<h2>Inccorect Rendering Case</h2>
<span>This count should be increased everysecond.</span>
<p>Count: {count}</p>
</div>
);
};
export default IncorrectRendering;
- Unpredictable Behavior: Stale closures can lead to unexpected and inconsistent behavior in React components. This might cause strange bugs that are difficult to identify and fix.
Example Code:
import React, { useState } from "react";
const UnpredictableBehavior: React.FC = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
// Incorrect: Stale closure capturing 'count'
setTimeout(() => {
setCount(count + 1);
}, 1000);
};
const decrementCount = () => {
// Incorrect: Stale closure capturing 'count'
setTimeout(() => {
setCount(count - 1);
}, 1000);
};
return (
<div>
<h2>Unpredictable Behavior Case</h2>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment Count in a second</button>
<button onClick={decrementCount}>Decrement Count in a second</button>
</div>
);
};
export default UnpredictableBehavior;
- Memory Leaks: In certain cases, stale closures can cause memory leaks. If a closure captures a reference to a component that has been unmounted, the component may not be properly garbage collected, leading to unnecessary memory consumption.
Example Code:
import React, { useState, useEffect } from "react";
const MemoryLeak: React.FC = () => {
const [count, setCount] = useState(0);
const [timerId, setTimerId] = useState<NodeJS.Timer | null>(null);
const startTimer = () => {
// Incorrect: Stale closure capturing 'count' and 'timerId'
const id = setInterval(() => {
console.log(`[Timer-${timerId}] Count is:${count}`);
setCount(count + 1);
}, 1000);
setTimerId(id);
};
const stopTimer = () => {
// Incorrect: Stale closure capturing 'timerId'
if (timerId !== null) {
clearInterval(timerId);
}
};
useEffect(() => {
return () => {
// Clean up the timer on component unmount
console.log("Unmount MemoryLeak!");
stopTimer();
};
}, []);
const handleButtonClick = () => {
startTimer();
};
return (
<div>
<h2>MemoryLeak Rendering Case</h2>
<p>
After clicking the button, the `setInterval` callback function is still being called even though this component has been unmounted.
</p>
<p>Count: {count}</p>
<button onClick={handleButtonClick}>Increment Count Every Second</button>
</div>
);
};
export default MemoryLeak;
How to Avoid Stale Closures in React:
To mitigate the impact of stale closures in React components, consider the following best practices:
-
Use the Dependency Array: When employing the useEffect
, useMemo ,
and useCallback
hook, always specify a dependency array as the second argument. This ensures that the effect only runs when the specified dependencies change, reducing the likelihood of stale closures.
-
Utilize Functional Updates: When updating state based on the previous state, use functional updates to prevent stale closures. For example, instead of setCount(count + 1)
, use setCount((prevCount) => prevCount + 1).
-
Properly Cleanup Effects: Always clean up any active subscriptions or timers in the useEffect
cleanup function. This helps avoid potential memory leaks that can be caused by stale closures.
Example Code:
import React, { useState, useEffect } from "react";
const BestPractice: React.FC = () => {
const [count, setCount] = useState(0);
// 1. List count as a dependency in the `useEffect` hook.
// So, it will recapture value once the `count` has changed
useEffect(() => {
const intervalId = setInterval(() => {
console.log("Count", count);
setCount(count + 1);
}, 1000);
// 3. Clear the interval on unmount to prevent memory leaks and unexpected behavior
return () => {
clearInterval(intervalId);
};
}, [count]);
const handleButtonClick = () => {
// 2. Use a functional update to prevent stale closures.
setCount((count) => count + 1);
};
return (
<div>
<p>{count}</p>
<button onClick={handleButtonClick}>Increase Counter</button>
</div>
);
};
export default BestPractice;
You can explore all the different use cases discussed in this article through the provided CodeSandbox project. It offers a hands-on learning experience, allowing you to experiment and gain a deeper understanding of the concepts.
Click on the following link to access the CodeSandbox project: https://codesandbox.io/s/stale-closure-issues-r69dcw
In conclusion, stale closures can be a subtle yet significant problem when working with asynchronous operations and React components. By understanding how closures capture variables and following best practices like using dependency arrays and functional updates, developers can reduce the occurrence of stale closures and ensure more consistent and predictable behaviour in their React applications.
Ready to eliminate the pitfalls of stale closures and optimize your React applications for consistent and predictable performance? Unlock the full potential of your code with our expert IT consulting services. Let us guide you through best practices, implement efficient solutions, and ensure a seamless experience in your asynchronous operations. Take the next step towards robust and reliable React development – consult with us today!
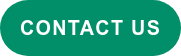