If you’re interested in developing a backend service with Rust language. There are many well-known and popular frameworks/open sources such as Warp, Rocket and Actix.
Why am I focusing on Actix?
Performance: this framework is designed for high performance and concurrency which means it can handle massive requests at the same time and can build a scalable web service.
Ergonomic: many open sources from Rust can provide great performance as well, but none make developers and maintainers feel as productive and comfortable as Actix. Actix is the one framework that can provide a clean structure and API that developers can use easily.
Ecosystem & Community: Actix is an active community where people can discuss and raise any issues.
The document of Actix is useful and readable to new beginners or experienced guys.
Starting with Cargo!!
Cargo is essential for Rust developers.
There are many features which cargo provides to use such as Dependency Management, Build System, Testing, Documentation and Publishing.
To begin, you must first install Rust on your computer, which can be done by following the installation instructions provided at https://www.rust-lang.org/tools/install.
Let’s see how to create and run a project
# create a project by using cargo command
cargo new simple-api
cd simple-api
# default folder structure
simple-api/
├── Cargo.toml
└── src/
└── main.rs
Here is an example Cargo.toml including Actix.
[package]
name = "simple-api"
version = "0.1.0"
edition = "2021"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
actix-web = "4"
serde = { version = "1.0", features = ["derive"] }
serde_json = {version = "1.0"}
My intention is to demonstrate how to create a controller for obtaining responses from a service in Actix.
# default folder structure that contains controller
simple-api/
├── Cargo.toml
└── src/
├── controller/
└── user/
└── user_controller.rs
└── user_struct.rs
└── main.rs
In user_struct.rs, you'll find conveniently organized structs that are utilized within the controller for ease of management.
use serde::Serialize;
#[derive(Serialize)]
pub struct User {
pub first_name: String,
pub last_name: String,
pub age: i16
}
#[derive(Serialize)]
pub struct UsersResponse {
pub status: String,
pub data: Vec<User>
}
Moving on to user_controller.rs
, This file contains the control logic for determining responses, and it includes a method for establishing the protocol in any incoming requests.
pub mod user_controller {
use actix_web::{get, web, Responder, Result};
use crate::controller::user::user_struct::{User, UsersResponse};
#[get("/users")]
async fn get_users() -> Result<impl Responder> {
let mut users: Vec<User> = Vec::new();
for index in 1..10 {
let first_name:String = "John ".to_string().to_owned() + &index.to_string().to_owned();
let user = User {
first_name,
last_name: "Doe".to_string(),
age: 20 + index
};
users.push(user);
}
let response = UsersResponse {
status: "ok".to_string(),
data: users
};
Ok(web::Json(response))
}
}
Final in main.rs
, we'll register a function from the controller and configure the application to serve it.
// register user_controller and user_struct
mod controller {
pub mod user {
pub mod user_controller;
pub mod user_struct;
}
}
// define for using get_users api
use crate::controller::user::user_controller::user_controller::get_users;
#[actix_web::main]
async fn main() -> std::io::Result<()> {
use actix_web::{App, HttpServer};
HttpServer::new(|| App::new().service(get_users)
)
.bind(("127.0.0.1", 8080))?
.run()
.await
}
Let’s see how to install the necessary dependencies and start serving our application.
# install dependencies
cargo build
# serve our application
cargo run
You can see the response from Actix by calling the API at localhost:8080/users.
{
"status": "ok",
"data": [
{
"first_name": "John 1",
"last_name": "Doe",
"age": 21
},
{
"first_name": "John 2",
"last_name": "Doe",
"age": 22
},
{
"first_name": "John 3",
"last_name": "Doe",
"age": 23
},
{
"first_name": "John 4",
"last_name": "Doe",
"age": 24
},
{
"first_name": "John 5",
"last_name": "Doe",
"age": 25
},
{
"first_name": "John 6",
"last_name": "Doe",
"age": 26
},
{
"first_name": "John 7",
"last_name": "Doe",
"age": 27
},
{
"first_name": "John 8",
"last_name": "Doe",
"age": 28
},
{
"first_name": "John 9",
"last_name": "Doe",
"age": 29
}
]
}
Conclusion
Discover the ease of implementing an API with Actix and Rust's reliable compiler that catches errors. Its clean structure and user-friendly features make it an ideal development choice. Ready to streamline your projects? Contact Us to explore our tailored IT consulting services today!
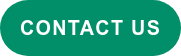