Before we begin, please ensure you’re ready to deliver an outstanding performance in Rust.
Little background
Rust language has been developed since 2006 by Mozilla. There’re a lot of open source frameworks which are used to implement backend services. for instance, Rocket, Warp and Actix Web. This article is focusing on basic things about Rust programming and syntax.
How to print in Rust??
fn main(){
println!("Hello Welcome From Rust");
}
Let’s see a variable declaration.
fn main(){
// maximum value of sign integer is from 2^(N-1) - 1, N = number of bits
// The maximum value is 2,147,483,647 (2^31 - 1)
// minimum value of sign integer is from -(2^(N-1)), N = number of bits
// The minimum value is -2,147,483,648 (-(2^31))
let signVariable: i32 = 30;
// maximum value of unsign integer is from 2^N - 1, N = number of bits
// The maximum value is 2^32 - 1 = 4,294,967,295
// The minimum value of unsign integer always is zero
let unsignVariable: u32 = 30;
// declaration of string
let stringVariable: String = String::from("Example String");
// if we declare variable without specified type
// the default type is i32 (sign integer 32bit)
let score = 100;
// 64-bit floating-point number
let floatingVariable: f64 = 3.14;
// declaration of boolean
let booleanVariable: bool = true;
}
Declaring the bit size of a variable in Rust can be beneficial for several reasons. First, it allows the compiler to optimize your code and make it more efficient. For example, if you know that you only need to store a value from 0 to 255, you can declare the variable as an 8-bit unsigned integer. This will save memory and make your code faster.
Second, Rust is designed to prevent unexpected side effects. This means that variables that are declared previously cannot be updated without the mut keyword. This helps to ensure that your code is safe and reliable.
Therefore, declaring both bit size and mutability of variables in Rust is a good practice that can help to make your code more efficient and safe.
fn main(){
// put mut in front of variable to make it as mutable
let mut editableInteger = 10;
editableInteger = 20;
// example, how to reassign string value
let mut editableString = String::from("Example String");
editableString = String::from("Hello Welcome");
}
Control flow statement
if-else if-else, statement is a common control flow statement that is used to execute different blocks of code based on the value of a condition. The syntax for this statement is similar in most programming languages, including Rust.
fn main() {
let number = 150;
if number > 20 && number < 200 {
println!("Number is greater than 20 and lower than 200");
} else if number == 200 {
println!("Number is equal to 200");
} else {
println!("Number is something else");
}
}
Loop
// example of while loop
let mut i = 0;
while i < 10 {
println!("hello");
i = i + 1;
}
// example of loop over a series of integers
let mut sum = 0;
for n in 1..11 {
sum += n;
}
Match (Switch case)
let x = 3;
match x {
1 => println!("x is 1"),
2 => println!("x is 2"),
3 => println!("x is 3"),
_ => println!("x is not 1, 2, or 3"),
}
Error and Reliability
Rust is a statically typed language, which means that the type of a variable must be known at compile time. This helps to prevent errors and make your code more reliable. For example, if you declare a variable as a String, you cannot update its value to an integer. The compiler will catch this error and prevent your code from running.
Conclusion
Ready to elevate your coding skills with Rust? For beginners familiar with other languages, transitioning is smooth. Let’s navigate Rust's key aspects together—like variable types and mutability—enhancing safety and performance.
Contact Us for tailored IT consulting and ace Rust programming!
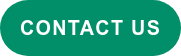